如何在React Native中从任意URL下载图像
React Native 中的图像下载
在这篇文章中,我们将了解如何在 React Native 中从任何 URL 下载图像。 对于这个任务,我尝试了许多不同的库和许多不同的方法,但发现使用下载图像 rn 获取 blob 图书馆很容易。
在搜索库解决方案下载文件时,我主要发现了 3 个库
- 反应本机获取 blob
- 反应本机FS
- rn 获取 blob
但你看 React-native-fetch-blob 与 React-native-fs 与 rn-fetch-blob 你会看到大多数人都在使用 rn-fetch-blob 的趋势,原因是
- 反应本机FS 是为 React Native 引入的第一个流行的文件系统管理库,但它们的原始创建者现在不再维护该库,它们突然发生了变化,新的维护者没有正确提供更新
- 反应本机获取 blob 是提供相同功能的替代解决方案,而react-native-fs没有维护者。
- rn 获取 blob 是的叉子 反应本机获取 blob 现在,react-native-fetch-blob 的维护者对该库进行了存档,并建议使用 rn-fetch-blob,因为与 react-native-fetch-blob 相比,它具有更多功能并进行了更新。
推荐:Outlook错误电子邮件系统在处理此邮件时出现问题
特点 rn 获取 blob
rn-fetch-blob 是一个 该项目致力于使 React Native 开发人员的文件访问和数据传输更加轻松、高效。 借助该库,您可以执行以下任务
- 直接从存储传输数据/向存储传输数据,无需 BASE64 桥接
- 文件 API 支持常规文件、Asset 文件和 CameraRoll 文件
- 原生到原生的文件操作API,减少JS桥接性能损失
- 文件流支持处理大文件
- Blob、File、XMLHttpRequest polyfills 使基于浏览器的库在 RN 中可用(实验性)
- JSON流支持基于 双簧管.js @吉姆希格森
下载图像
要使用 rn-fetch-blob 下载图像,我们将使用 RNFetchBlob 组件,该组件提供具有一些不同配置的 fetch 方法。 这是下载图像的代码片段。
const { config, fs } = RNFetchBlob;
let PictureDir = fs.dirs.PictureDir;
let options = {
fileCache: true,
addAndroidDownloads: {
//Related to the Android only
useDownloadManager: true,
notification: true,
path:
PictureDir +
'/image_' +
Math.floor(date.getTime() +
date.getSeconds() / 2) + ext,
description: 'Image',
},
};
config(options)
.fetch('GET', image_URL)
.then(res => {
//Showing alert after successful downloading
console.log('res -> ', JSON.stringify(res));
alert('Image Downloaded Successfully.');
});
项目概况
在此示例中,我们将有一个图像组件,用于显示我们要下载的图像和一个开始下载过程的按钮。 单击按钮后,我们将检查平台
- 如果找到Android,那么我们将请求许可,一旦获得许可,我们将开始下载图像
- 如果找到iOS我们将直接开始下载
我们将下载的图片存放到设备的默认图片目录中。 我希望您能明白我的意思,所以让我们立即查看代码。
制作 React Native 应用程序
React Native 入门将帮助您更多地了解如何制作 React Native 项目。 我们将使用 React Native 命令行界面来制作我们的 React Native 应用程序。
如果您之前安装了全局的react-native-cli软件包,请将其删除,因为它可能会导致意外问题:
npm uninstall -g react-native-cli @react-native-community/cli
运行以下命令创建一个新的 React Native 项目
npx react-native init ProjectName
如果你想使用特定的 React Native 版本启动一个新项目,你可以使用 –version 参数:
npx react-native init ProjectName --version X.XX.X
注意如果上述命令失败,您可能使用的是旧版本 react-native
或者 react-native-cli
在您的电脑上全局安装。 尝试卸载 cli 并使用 npx 运行 cli。
这将在项目目录中创建一个带有名为 App.js 的索引文件的项目结构。
安装依赖
正如我所说,我们将使用 rn-fetch-blob
需要在我们的项目中安装的库并安装该库,打开终端并跳转到您的项目
cd ProjectName
并运行以下命令
npm install rn-fetch-blob --save
CocoaPods 安装
现在我们需要安装 pod
cd ios && pod install && cd ..
Android 访问联系人列表的权限
在此示例中,我们将把下载的文件存储在外部存储中,为此我们必须向 AndroidManifest.xml
文件。 请在 AndroidMnifest.xml 中添加以下权限。
去 YourProject -> android -> app -> main -> AndroidMnifest.xml
<uses-permission
android:name="android.permission.WRITE_EXTERNAL_STORAGE"
/>
允许 | 目的 |
---|---|
写外部存储 | 在外部存储中创建任何文件 |
有关权限的更多信息,您可以查看这篇文章。
在 React Native 中下载图像的代码
在任何代码编辑器中打开 App.js 并将代码替换为以下代码
应用程序.js
// How to Download an Image in React Native from any URL
// https://aboutreact.com/download-image-in-react-native/
// Import React
import React from 'react';
// Import Required Components
import {
StyleSheet,
Text,
View,
TouchableOpacity,
PermissionsAndroid,
Image,
Platform,
} from 'react-native';
// Import RNFetchBlob for the file download
import RNFetchBlob from 'rn-fetch-blob';
const App = () => {
const REMOTE_IMAGE_PATH =
'https://raw.githubusercontent.com/AboutReact/sampleresource/master/gift.png'
const checkPermission = async () => {
// Function to check the platform
// If iOS then start downloading
// If Android then ask for permission
if (Platform.OS === 'ios') {
downloadImage();
} else {
try {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.WRITE_EXTERNAL_STORAGE,
{
title: 'Storage Permission Required',
message:
'App needs access to your storage to download Photos',
}
);
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
// Once user grant the permission start downloading
console.log('Storage Permission Granted.');
downloadImage();
} else {
// If permission denied then show alert
alert('Storage Permission Not Granted');
}
} catch (err) {
// To handle permission related exception
console.warn(err);
}
}
};
const downloadImage = () => {
// Main function to download the image
// To add the time suffix in filename
let date = new Date();
// Image URL which we want to download
let image_URL = REMOTE_IMAGE_PATH;
// Getting the extention of the file
let ext = getExtention(image_URL);
ext="." + ext(0);
// Get config and fs from RNFetchBlob
// config: To pass the downloading related options
// fs: Directory path where we want our image to download
const { config, fs } = RNFetchBlob;
let PictureDir = fs.dirs.PictureDir;
let options = {
fileCache: true,
addAndroidDownloads: {
// Related to the Android only
useDownloadManager: true,
notification: true,
path:
PictureDir +
'/image_' +
Math.floor(date.getTime() + date.getSeconds() / 2) +
ext,
description: 'Image',
},
};
config(options)
.fetch('GET', image_URL)
.then(res => {
// Showing alert after successful downloading
console.log('res -> ', JSON.stringify(res));
alert('Image Downloaded Successfully.');
});
};
const getExtention = filename => {
// To get the file extension
return /(.)/.exec(filename) ?
/(^.)+$/.exec(filename) : undefined;
};
return (
<View style={styles.container}>
<View style={{ alignItems: 'center' }}>
<Text style={{ fontSize: 30, textAlign: 'center' }}>
React Native Image Download Example
</Text>
<Text
style={{
fontSize: 25,
marginTop: 20,
marginBottom: 30,
textAlign: 'center',
}}>
www.aboutreact.com
</Text>
</View>
<Image
source={{
uri: REMOTE_IMAGE_PATH,
}}
style={{
width: '100%',
height: 100,
resizeMode: 'contain',
margin: 5
}}
/>
<TouchableOpacity
style={styles.button}
onPress={checkPermission}>
<Text style={styles.text}>
Download Image
</Text>
</TouchableOpacity>
</View>
);
};
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
button: {
width: '80%',
padding: 10,
backgroundColor: 'orange',
margin: 10,
},
text: {
color: '#fff',
fontSize: 20,
textAlign: 'center',
padding: 5,
},
});
运行 React Native 应用程序
再次打开终端并使用跳转到您的项目。
cd ProjectName
1.启动Metro Bundler
首先,您需要启动 Metro,React Native 附带的 JavaScript 捆绑器。 要启动 Metro 捆绑程序,请运行以下命令
npx react-native start
一旦您启动 Metro Bundler,它将永远在您的终端上运行,直到您将其关闭。 让 Metro Bundler 在自己的终端中运行。 打开一个新终端并运行该应用程序。
2.启动React Native应用程序
在 Android 虚拟设备或真实调试设备上运行项目
npx react-native run-android
或在 iOS 模拟器上运行(仅限 macOS)
npx react-native run-ios
输出截图
安卓
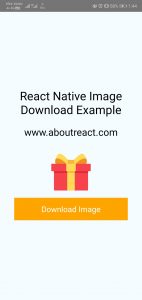
iOS系统
这是如何在 React Native 中从任何 URL 下载图像的方法。 如果您有任何疑问或想分享有关该主题的内容,您可以在下面发表评论或在此处联系我们。 很快就会有更多帖子发布。